numpy数组也就是ndarray,它的本质是一个对象,那么一定具有一些对象描述的属性,同时,它还有元素,其元素也有一些属性。本节主要介绍ndarray以及其元素的属性和属性的操作。
1. ndarray的属性
ndarray有两个属性:维度(ndim)和每个维度的大小shape(也就是每个维度元素的个数)
import numpy as np
a = np.arange(24)
a.shape=(2,3,4)
print('数组为:', a)
print('数组的维度:', a.ndim)
print('数组维度的大小',a.shape)
输出:
数组为: [[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]]
[[12 13 14 15]
[16 17 18 19]
[20 21 22 23]]]
数组的维度: 3
数组维度的大小 (2, 3, 4)
[/code]
对于ndarray数组的属性的操作只能操作其shape,也就是每个维度的个数,同时也就改变了维度(shape是一个元组,它的长度就是维度(ndim)),下面介绍两种改变数组shape的方式:
```code
import numpy as np
a = np.arange(24)
a.shape=(2,3,4)
# a.shape=(4,6),直接对a进行操作
a.shape = (4,6)
print('a:',a)
#a.reshape(3,8)是返回一个修改后维度大小的新数组,不会修改原来的数组a
b = a.reshape(3,8)
print('b:',b)
[/code]
```code
输出:
a: [[ 0 1 2 3 4 5]
[ 6 7 8 9 10 11]
[12 13 14 15 16 17]
[18 19 20 21 22 23]]
b: [[ 0 1 2 3 4 5 6 7]
[ 8 9 10 11 12 13 14 15]
[16 17 18 19 20 21 22 23]]
[/code]
**notes:**
1.对shape直接赋值的方式是修改原数组的属性,没有返回新的数组。reshape的方式不会修改原数组的属性,一定会返回一个新的数
组。
2. 修改属性的时候,属性元素之和一定要等于数组的元素之和,例如原数组有24个元素,则属性只能修改为:一维:(24,)二维:(2,12)、(3,8)、(4,6),三维:(2,3,4),四维:(2,3,2,2)等
# 2. ndarray元素的属性
1. 单个元素所占存储的字节数:itemsize
2. 其他属性:flags
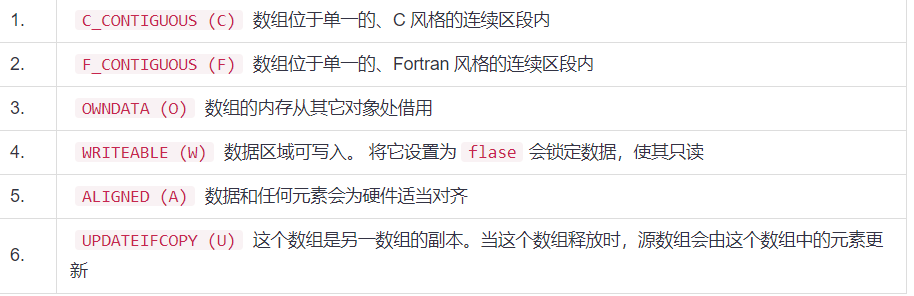
```code
import numpy as np
a = np.arange(24)
a.shape=(2,3,4)
print('单个元素所占字节数:',a.itemsize)
print('元素其他属性:',a.flags)
[/code]
```code
输出:
单个元素所占字节数: 4
元素其他属性: C_CONTIGUOUS : True
F_CONTIGUOUS : False
OWNDATA : True
WRITEABLE : True
ALIGNED : True
WRITEBACKIFCOPY : False
UPDATEIFCOPY : False
[/code]
# 3. ndarray元素的类型
```code
import numpy as np
a = np.arange(24)
a.shape=(2,3,4)
print('元素的类型',a.dtype)
# 对dtype直接复制是直接在原数组上修改的方式
a.dtype= 'float32'
print('修改后的元素的类型',a.dtype)
# astype不会修改原数组的类型,会返回一个新的数组
b=a.astype('int32')
print('修改后a的元素的类型',a.dtype)
print('修改后b的元素的类型',b.dtype)
[/code]
```code
元素的类型 int32
修改后的元素的类型 float32
修改后a的元素的类型 float32
修改后b的元素的类型 int32
[/code]
**notes:** 注意dtype直接复制与astype的区别
